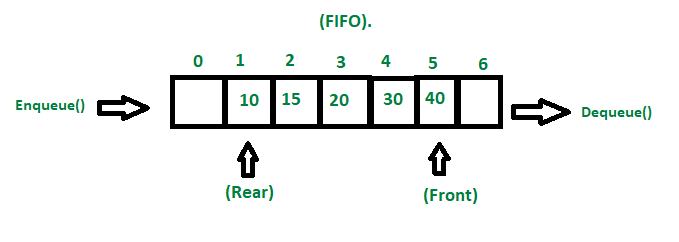
What is Queue?
A queue is a linear data structure that is open at both ends and the operations are performed in First In First Out (FIFO) order.
We define a queue to be a list in which all additions to the list are made at one end, and all deletions from the list are made at the other end. The element which is first pushed into the order, the operation is first performed on that.
FIFO Principle of Queue:
- A Queue is like a line waiting to purchase tickets, where the first person in line is the first person served. (i.e. First come first serve).
- Position of the entry in a queue ready to be served, that is, the first entry that will be removed from the queue, is called the front of the queue(sometimes, head of the queue), similarly, the position of the last entry in the queue, that is, the one most recently added, is called the rear (or the tail) of the queue. See the below figure.
FIFO property of queue
Characteristics of Queue:
- Queue can handle multiple data.
- We can access both ends.
- They are fast and flexible.
Queue Representation:
Array Representation:
Like stacks, Queues can also be represented in an array: In this representation, the Queue is implemented using the array. Variables used in this case are
- Queue: the name of the array storing queue elements.
- Front: the index where the first element is stored in the array representing the queue.
- Rear: the index where the last element is stored in an array representing the queue.
Array representation of queue:
C++
|
Linked List Representation:
A queue can also be represented in Linked-lists, Pointers, and Structures.
Linked-list representation of Queue:
C++
|
Types of Queue:
There are different types of queue:
Input Restricted Queue
This is a simple queue. In this type of queue, the input can be taken from only one end but deletion can be done from any of the ends.
Output Restricted Queue
This is also a simple queue. In this type of queue, the input can be taken from both ends but deletion can be done from only one end.
Circular Queue
This is a special type of queue where the last position is connected back to the first position. Here also the operations are performed in FIFO order.
Double-Ended Queue (Deque)
In a double-ended queue the insertion and deletion operations, both can be performed from both ends.
Priority Queue
A priority queue is a special queue where the elements are accessed based on the priority assigned to them.
To learn more about different types of queues, read the article on “Types of Queues“.
Basic Operations of Queue:
There are few supporting operations (auxiliary operations):
front():
This operation returns the element at the front end without removing it.
C++
|
rear():
This operation returns the element at the rear end without removing it.
C++
|
isEmpty():
This operation returns a boolean value that indicates whether the queue is empty or not.
C++
|
isFull():
This operation returns a boolean value that indicates whether the queue is full or not.
C++
|
Enqueue():
Adds (or stores) an element to the end of the queue.
The following steps should be taken to enqueue (insert) data into a queue:
- Step 1: Check if the queue is full.
- Step 2: If the queue is full, return overflow error and exit.
- Step 3: If the queue is not full, increment the rear pointer to point to the next empty space.
- Step 4: Add the data element to the queue location, where the rear is pointing.
- Step 5: return success.
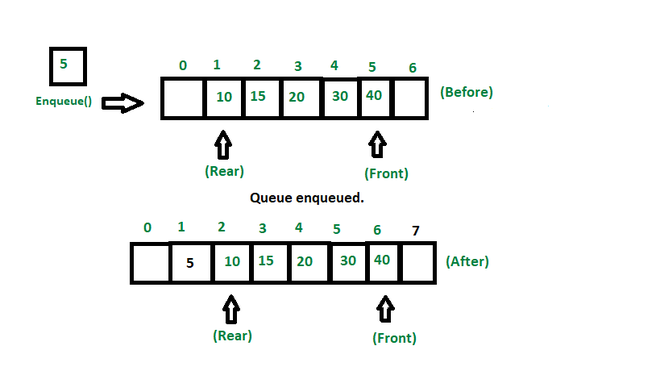
Enqueue representation
Implementation of Enqueue:
C++
|
Dequeue():
Removes (or access) the first element from the queue.
The following steps are taken to perform the dequeue operation:
- Step 1: Check if the queue is empty.
- Step 2: If the queue is empty, return the underflow error and exit.
- Step 3: If the queue is not empty, access the data where the front is pointing.
- Step 4: Increment the front pointer to point to the next available data element.
- Step 5: The Return success.
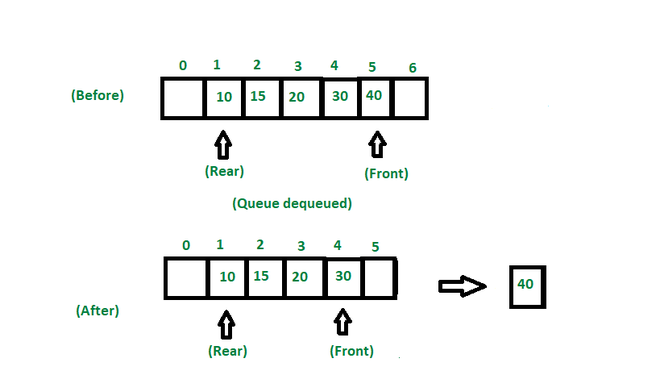
Dequeue operation
Implementation of dequeue:
C++
|
Implementation of queue:
Below is an implementation of all the operations used in a queue.
C++
|
10 enqueued to queue 20 enqueued to queue 30 enqueued to queue 40 enqueued to queue 10 dequeued from queue Front item is 20 Rear item is 40
Time complexity: All the operations have O(1) time complexity.
Auxiliary Space: O(N)
Applications of Queue:
Application of queue is common. In a computer system, there may be queues of tasks waiting for the printer, for access to disk storage, or even in a time-sharing system, for use of the CPU. Within a single program, there may be multiple requests to be kept in a queue, or one task may create other tasks, which must be done in turn by keeping them in a queue.
- It has a single resource and multiple consumers.
- It synchronizes between slow and fast devices.
- In a network, a queue is used in devices such as a router/switch and mail queue.
- Variations: dequeue, priority queue and double-ended priority queue.
FAQs (Frequently asked questions):
1. What data structure can be used to implement a priority queue?
Priority queues can be implemented using a variety of data structures, including linked lists, arrays, binary search trees, and heaps. Priority queues are best implemented using the heap data structure.
2. Queues are used for what purpose?
In addition to making your data persistent, queues reduce errors that occur when different parts of your system are down.
3. In data structures, what is a double-ended queue?
In a double-ended queue, elements can be inserted and removed at both ends.
4. What is better, a stack or a queue?
If you want things to come out in the order you put them in, use a queue. Stacks are useful when you want to reorder things after putting them in.
Related articles: