Multidimensional arrays are arrays that have more than one dimension. For example, a simple array is a 1-D array, a matrix is a 2-D array, and a cube or cuboid is a 3-D array but how to visualize arrays with more than 3 dimensions, and how to iterate over elements of these arrays? It is simple, just think of any multidimensional array as a collection of arrays of lower dimensions.
n-D array = Collection of (n-1)D arrays
For example, a matrix or 2-D array is a collection of 1-D arrays.
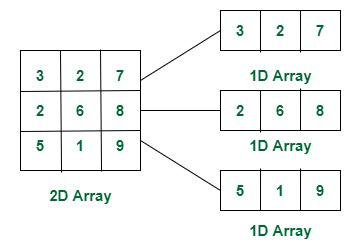
2D Array is a collection of 1D Arrays
Similarly, you can visualize 3-D arrays and other multidimensional arrays.
How to iterate over elements of a Multidimensional array?
It can be observed that only a 1-D array contains elements and a multidimensional array contains smaller dimension arrays.
- Hence first iterate over the smaller dimension array and iterate over the 1-D array inside it.
- Doing this for the whole multidimensional array will iterate over all elements of the multidimensional array.
Example 1: Iterating over a 2-D array
C++
|
3 2 7 2 6 8 5 1 9
Example 2: Iterating over a 3D array
C++
|
Inside 1 2D array in 3-D array Inside 1 1D array of the 2-D array 1 2 Inside 2 1D array of the 2-D array 3 4 Inside 2 2D array in 3-D array Inside 1 1D array of the 2-D array 5 6 Inside 2 1D array of the 2-D array 7 8